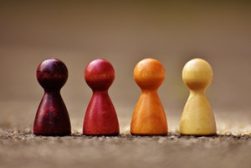
“Sarcasm is the last refuge of the imaginatively bankrupt.”
― Cassandra Clare, City of Bones
The legendary “The Muppet Show” featured two older characters, Statler and Waldorf, who sat next to each other in a balcony, always nagging and cracking sarcastic jokes at the expense of others. On various projects, I’ve come across developers who behaved just like them.
Statlers and Waldorfs are typically found in medium-to-large corporations that have been around for quite some time—just like the Statlers and Waldorfs themselves, they usually have a long history with their company.
APPEARANCE
The Statlers and Waldorfs that I’ve met were always male and clearly above age 40, some of them way into their 50s. They didn’t care much for looks and grooming and were often overweight.
But looks can be deceiving. What really defines Statlers and Waldorfs is not their looks, but rather their mental attitude. There are Statlers and Waldorfs that don’t fit the description above but who are still who they are, even at age 28. In my experience, there are “classic” Waldorf and Statler types and “concealed” Waldorf and Statler types. Mental attitude and looks only coincide in the former type.
PERSONALITY TRAITS
Regardless of their age or physical appearance, their mental age is above 70 and even if they show up in the office every day, they retired mentally a long time ago.
At their core, Statlers and Waldorfs are extremely dissatisfied. About what and why this is the case, we don’t know and are unlikely to ever find out. It’s possible that they were once positively enthusiastic about their craft but got severely hurt or disappointed along their way.
All Statlers and Waldorfs have in common that they avoid change like the plague. At first, this comes as a bit of surprise, as one might think that dissatisfied people would happily welcome new approaches, ideas, and tools. Not so for the Statlers and Waldorfs of the world. They’d rather stay miserable for the rest of their lives than give something new a chance.
Unable to accept change in an ever-changing world, the Statlers and Waldorfs become bitter and resentful and express it through endless streams of sarcasm. High-quality production code, however, only trickles out of them—at best. If you give them a task, chances are that they will complain forever about missing requirements, missing documentation, inferior tools, and lack of support from others.
TOOLING
Tools are not highly valued by Statlers and Waldorfs. They use the tools everyone else uses and when asked to pick a programming language, they reach for the top of the TIOBE index; that is, Java or C. If they pick Java, they don’t use any of the features that came out after JDK1.1; should they pick C, K&R C is more than sufficient for them.
CASE IN POINT
I vividly remember the day when I presented a wonderful idea to a Statler/Waldorf type of colleague early in my career. I was so thrilled, so sure that it was a big hit and naively assumed that everyone else was likewise excited. But boy, was I mistaken! Before I had even finished telling my story, the Uber-Statler/Waldorf—with a big smirk on his face—started firing dozens of poisoned darts at me, why it would never work, why it was a ridiculous idea in the first place, and so on. As you can imagine, my motivation dropped to the floor. Days later, I implemented my idea nevertheless. With great success, as it turned out. However, it took days until the Statler/Waldorf finally stopped mocking me.
ADVICE
I’m personally convinced that it’s impossible to reprogram the firmware (or rather wetware) of a Statler/Waldorf; yet, I’m hoping that the following tips help reduce the likelihood of you turning into a Statler/Waldorf zombie yourself:
1. Don’t look old. No, you don’t have to become a Programming Hipster, rather try to blend in with the rest of the team. If you are the only one wearing Birkenstocks, get rid of them. Get rid of those highwater cords and suspenders, too.
2. Don’t talk old. Naturally, as an experienced developer you have seen a lot—good things and bad things. But trust me: nobody wants to hear that story where you implemented toaster control software in 256 bytes of ROM at GE in the late 1970s—over and over again. Would you trust a doctor that pops up at your door and tells you that he has the perfect medical treatment for you? No? That’s why you should never give unsolicited advice, either. Wait until they come to you. Bite your tongue. Most importantly, stop being sarcastic; sarcasm only hurts and is a sign of weakness, not strength.
3. Don’t think old. Over the years, you have probably learned that most problems that projects face these days are not technological but rather sociological in nature. The kind of people and the way they work together has much more influence on the overall success than the choice of programming language. Nevertheless, don’t fight it if the majority of the team wants to give a hip programming language a try on the next project. Trying out new things boosts motivation and extends the knowledge portfolio. Just like life itself, software development is a continuous learning process. Remember the quote from Steve Siebold: “You’re either growing or dying. Stagnation does not exist in the universe.”
RATING
According to the Q²S² framework, a Statler/Waldorf’s rating is 2/2/2/1.
CONCLUSION
Some people build, and some destroy—a fact that can be easily observed by watching children playing with Lego bricks. Waldorf and Statlers are destructive rather than constructive. Maybe they excel as software risk managers; I can imagine that they come up with risks that nobody else has ever thought of. Or perhaps they should work in the testing department where they will use their negativity to find even the most unthinkable bugs. But whatever you do—keep them as far away from your developers as possible.